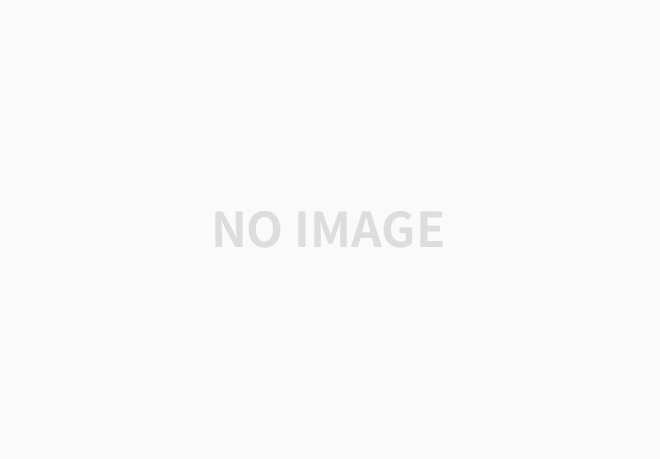
private void button1_Click(object sender, EventArgs e)
{
// 초기 디렉토리 설정
openFileDialog1.InitialDirectory = "C:\\";
// 파일 필터 설정
openFileDialog1.Filter = "모든 파일 (*.*)|*.*|텍스트 파일 (*.txt)|*.txt|바이너리 파일 (*.bin)|*.bin|데이터 파일 (*.dat)|*.dat";
// 마지막으로 파일 대화 상자에서 선택한 디렉토리를 기억하고, 다음번 파일 대화 상자를 열 때에는 이전에 선택한 디렉토리를 자동으로 선택
openFileDialog1.RestoreDirectory = true;
if (openFileDialog1.ShowDialog() == DialogResult.OK)
{
// 파일 경로를 TextBox에 표시
textBox1.Text = openFileDialog1.FileName;
}
}
private void button3_Click(object sender, EventArgs e)
{
this.textBox2.Text = "";
}
private uint Crc32(byte[] data)
{
uint crc = 0xFFFFFFFF;
for (int i = 0; i < data.Length; i++)
{
byte b = data[i];
crc ^= b;
for (int j = 0; j < 8; j++)
{
if ((crc & 1) != 0)
{
crc = (crc >> 1) ^ 0xEDB88320;
}
else
{
crc = crc >> 1;
}
}
}
return crc ^ 0xFFFFFFFF;
}
private void button2_Click(object sender, EventArgs e)
{
// 파일 경로 가져오기
string filePath = textBox1.Text;
if (File.Exists(filePath))
{
// 파일의 모든 바이트를 읽어서 바이트 배열로 반환. 파일의 크기가 큰 경우 시간이 걸릴 수 있음
byte[] fileBytes = File.ReadAllBytes(filePath);
this.textBox2.Text = "0x" + Crc32(fileBytes).ToString("X8");
}
}
'프로그래밍 > C# (WinForms)' 카테고리의 다른 글
WinForms ] Anchor, Dock properties (0) | 2023.05.14 |
---|---|
C#, WinForms ] Event 와 delegate (0) | 2023.05.14 |
C#, WinForms ] Drag & Drop 으로 파일 경로 얻기 (2) | 2023.05.14 |
C# ] delegate (0) | 2023.05.14 |
C#, WinForms ] 폼 시작시, 종료시 이벤트 호출 순서 (0) | 2023.05.14 |