filesystem 라이브러리는 파일 및 디렉토리 작업을 단순화하고 일반화하는 기능을 제공한다.
C++ 17 표준 부터 포함되었으며, gcc/g++ 8 이상 최신 컴파일러에서 지원된다.
experimental/filesystem 헤더를 포함하면 이전 버전에서 사용할 수 있을 수도 있다.
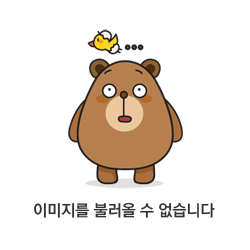
📂 현재 경로 출력
#include <iostream>
#include <filesystem>
using namespace std;
namespace fs = filesystem;
int main() {
fs::path currentPath = fs::current_path();
cout<< "Current Path: " << currentPath << endl;
return 0;
}
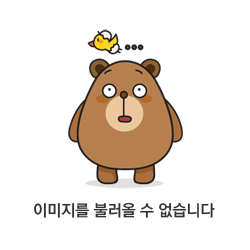
📂 디렉터리 탐색, 파일/디렉터리 구분
#include <iostream>
#include <filesystem>
using namespace std;
namespace fs = filesystem;
int main() {
fs::path directoryPath("Debug");
for (const auto& entry : fs::directory_iterator(directoryPath)) {
if (entry.is_regular_file()) {
cout << "File: " << entry.path() << endl;
}
else if (entry.is_directory()) {
cout << "Directory: " << entry.path() << endl;
}
}
return 0;
}
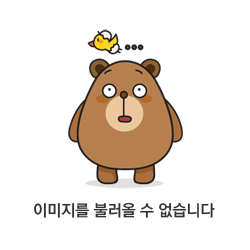
📂 파일 이동/복사
#include <iostream>
#include <filesystem>
using namespace std;
namespace fs = filesystem;
int main() {
fs::path sourceFile("Debug/formatHex.exe");
fs::path destinationFile("formatHex.exe");
try {
fs::rename(sourceFile, destinationFile);
cout << "File moved successfully." << endl;
}
catch (const fs::filesystem_error& e) {
cout << "Failed to move file: " << e.what() << endl;
}
try {
fs::copy(destinationFile, sourceFile);
cout << "File copied successfully." << endl;
}
catch (const std::filesystem::filesystem_error& e) {
cout << "Failed to copy file: " << e.what() << endl;
}
return 0;
}
📂 디렉터리 생성
#include <iostream>
#include <filesystem>
using namespace std;
namespace fs = filesystem;
int main() {
fs::path dirPath("Debug2");
if (fs::create_directory(dirPath)) {
cout << "Directory created successfully." << endl;
}
else {
cout << "Failed to create directory." << endl;
}
return 0;
}
📂 경로에 파일 존재여부 확인
#include <iostream>
#include <filesystem>
using namespace std;
namespace fs = filesystem;
int main() {
fs::path filePath("path/something.txt");
if (fs::exists(filePath)) {
cout << "File exists." << endl;
}
else {
cout << "File does not exist." << endl;
}
return 0;
}
'프로그래밍 > C++' 카테고리의 다른 글
C++ ] static_cast와 reinterpret_cast. 그 외 const_cast, dynamic_cast (0) | 2023.05.28 |
---|---|
C++ ] 예외처리 try-catch와 throw (0) | 2023.05.28 |
C++ ] JSON 데이터 포맷, rapidjson 라이브러리 사용해 파싱 (0) | 2023.05.14 |
C++ ] stream 상속관계 (0) | 2023.05.14 |
C++ ] accumulate 함수 사용시 주의사항 (0) | 2023.03.31 |